The 1.3 inch OLED display is very popular in the Arduino world due to its features and versatility. Here are some of the most important features summarized:
- Screen technology: OLED (Organic Light Emitting Diode) offers self-illuminating pixels that do not require background light. This makes the displays very thin and light.
- Screen size: 1.3 inch diagonal, which corresponds to a screen area of approx. 33.02 mm2.
- Resolution: Typically 128×64 pixels, which provides good clarity and detail for small projects.
- Contrast and brightness: Very high contrast (typically >10,000:1), as OLEDs can display true black. This results in sharp and vivid images.
- Energy consumption: Low energy consumption, especially when displaying black pixels, as unlit pixels do not consume any power.
- Control: Supports multiple communication protocols, such as I2C and SPI, which facilitates integration into various projects.
- Viewing angle: Very wide viewing angle (>160 degrees), which makes it easier to read from different positions.
- Service life: Long service life of the OLED elements, typically over 20,000 hours.
- Libraries and support: There are numerous libraries and community support for use with Arduino (e.g. Adafruit GFX and Adafruit SSD1306).
- Versatility: Ideal for many applications such as displaying sensor values, clocks, user interfaces and more. Brightness can be controlled by software. Compatible with many microcontrollers, including Arduino, ESP8266, and ESP32.
These features make the 1.3 inch OLED display an excellent choice for many Arduino projects where a clear and low-power display is required.
Setting up the circuit with the ESP32
Parts required:
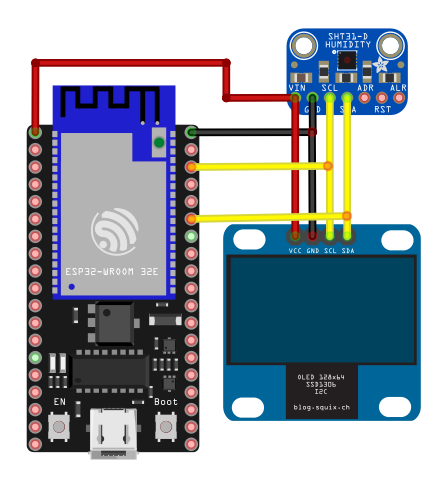
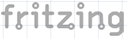
As we are also using the I2C bus here, we only need to connect the two data lines in parallel to those of the ESP32 or SHT31.
Programming with VS Studio Code
We need 2 libraries for programming the display.
the Adafrueit_GFX.h and the Adafrueit_SSD1306.h.
You can find these as usual under Libraries in PIO Home.
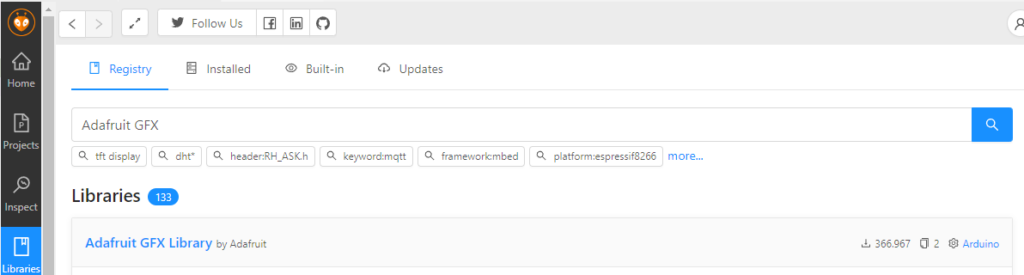
As soon as both libraries are installed, your platform.ini should look like this:
[env:esp32dev]
platform = espressif32
board = esp32dev
framework = arduino
lib_deps =
adafruit/Adafruit GFX Library@^1.11.9
adafruit/Adafruit SSD1306@^2.5.10
adafruit/Adafruit SHT31 Library@^2.2.2
monitor_speed=115200
Let’s turn to the code
#include <Wire.h> #include <Adafruit_GFX.h> #include <Adafruit_SSD1306.h> #include <Adafruit_SHT31.h> // OLED display width and height for 0.96 or 1.3 inch display #define SCREEN_WIDTH 128 #define SCREEN_HEIGHT 64 // Create an SSD1306 display instance Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, -1); // Creating an SHT31 sensor instance Adafruit_SHT31 sht31 = Adafruit_SHT31(); void setup() { // Starting serial communication Serial.begin(115200); // Check whether the display can be initialized if(!display.begin(SSD1306_SWITCHCAPVCC, 0x3C)) { Serial.println(F("SSD1306-Initialization failed")); for(;;); } // Clear the buffer display.clearDisplay(); // Text formatieren display.setTextSize(1); // Text size display.setTextColor(SSD1306_WHITE); // Text color // Display welcome text display.setCursor(0,0); // Place cursor at the start display.println(F("DIYTechAdventure")); display.display(); delay(2000); // Pause for 2 seconds // Check whether the sensor can be initialized if (!sht31.begin(0x44)) { // I2C Adresse für SHT31 Serial.println("SHT31-Initialization failed"); while (1) delay(1); } } void loop() { float temperature = sht31.readTemperature(); float humidity = sht31.readHumidity(); if (!isnan(temperature)) { // Check whether the temperature measurement was successful Serial.print("Temperature: "); Serial.print(temperature); Serial.println(" *C"); } else { Serial.println("Error when reading the temperature"); } if (!isnan(humidity)) { // Check whether the moisture measurement was successful Serial.print("Humidity: "); Serial.print(humidity); Serial.println(" %"); } else { Serial.println("Error when reading the humidity"); } // Update display display.clearDisplay(); display.setCursor(0, 0); display.println(F("DIYTechAdventure")); display.setTextSize(1); display.setCursor(0, 20); display.print("Temp: "); display.print(temperature); display.println(" C"); display.setCursor(0, 40); display.print("Humidity: "); display.print(humidity); display.println(" %"); display.display(); delay(10000); // Pause for 10 seconds }
Here are a few explanations of the individual parts of the code.
As mentioned above, the required libraries must first be integrated.
#include <Wire.h> #include <Adafruit_GFX.h> #include <Adafruit_SSD1306.h> #include <Adafruit_SHT31.h>
// The number of pixels for the width and height of the screen are agreed here #define SCREEN_WIDTH 128 #define SCREEN_HEIGHT 64
The instances for display and sensor are then created.
The line Adafruit_SSD1306 Display(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, -1); initializes the display. The parameter -1 is used to indicate that no reset pin is used. If you have a display without a special reset pin or the reset pin is not connected, you can set this parameter to -1. If your display has a reset pin and it is connected, you would enter the pin number here.
// Create a SSD1306 display instance Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, -1); // Create a SHT31 sensor instance Adafruit_SHT31 sht31 = Adafruit_SHT31();
- for: The key word for a loop.
- (;;): The head of the bow, which normally consists of three parts:
- Initialization: Normally an expression that is executed once before the loop starts. Here it is empty.
- Condition: An expression that is checked before each loop pass. If it is true, the loop body is executed. If it is false, the loop ends. Here it is empty, which means that the loop is always true.
- Iteration: An expression that is executed after each loop pass. Here it is empty.
As all three parts are empty, the loop is executed endlessly.
// Check that the display can be initialized if(!display.begin(SSD1306_SWITCHCAPVCC, 0x3C)) { Serial.println(F("SSD1306-Initialization failed")); for(;;);
In the loop you will see the reading of temperature and humidity, which has already been described here. Then it’s time to write to the display.
.clearDisplay() clears the display on the screen
.setCursor sets the cursor to the top left position. Column 1, line 1
.println writes my text “DIYTechAdventure”
.setTextSize – sets the font size to 1
.setCursor(0,20) Move the cursor to the far left of the screen and 20 pixels down.
The other lines also repeat the process for the humidity display.
In the loop you will see the reading of temperature and humidity, which has already been described here. Then it’s time to write to the display.
display.display() – then writes on the display
// Update display display.clearDisplay(); display.setCursor(0, 0); display.println(F("DIYTechAdventure")); display.setTextSize(1); display.setCursor(0, 20); display.print("Temp: "); display.print(temperature); display.println(" C"); display.setCursor(0, 40); display.print("Humidity: "); display.print(humidity); display.println(" %"); display.display();
And here we see the result of our efforts. As specified in the code, the display is updated every 10 seconds.
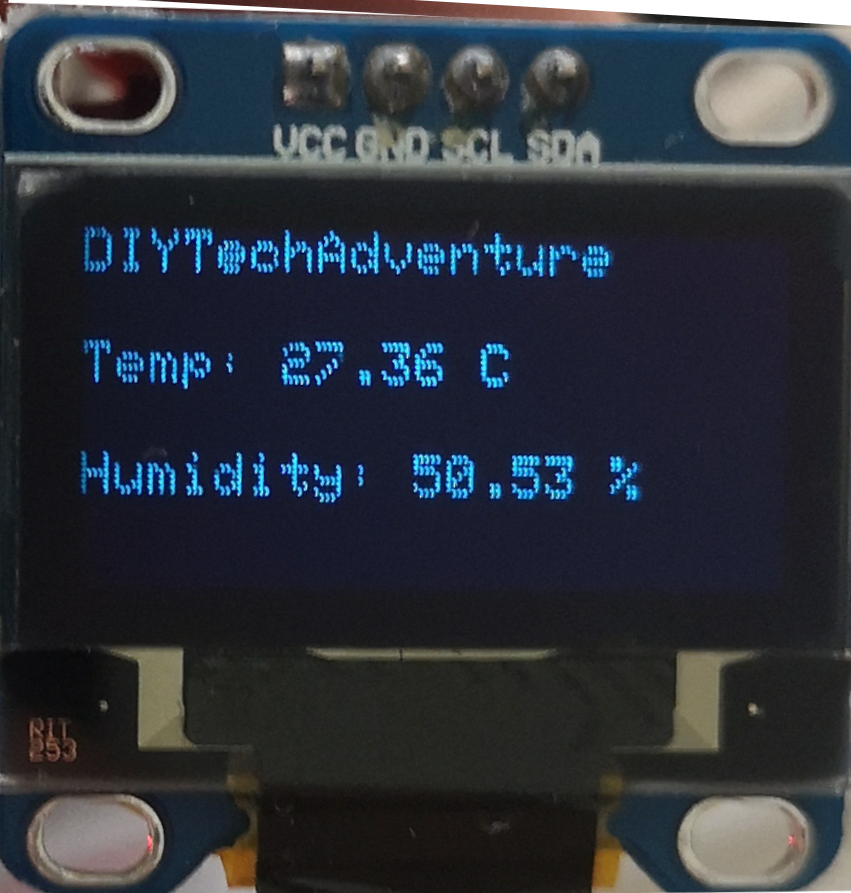
I hope you were able to achieve similar results with these with DIYTechAdventure. Look forward to more exciting adventures and let me know which sensors you would like to use for the temperature and humidity measurements.