The pin assignment of the ESP32_Devkit_V with 38 PINs is shown here. For the sake of clarity, this is a simplified representation. As a rule, many PINs are assigned more than once. I will go into this in more detail.
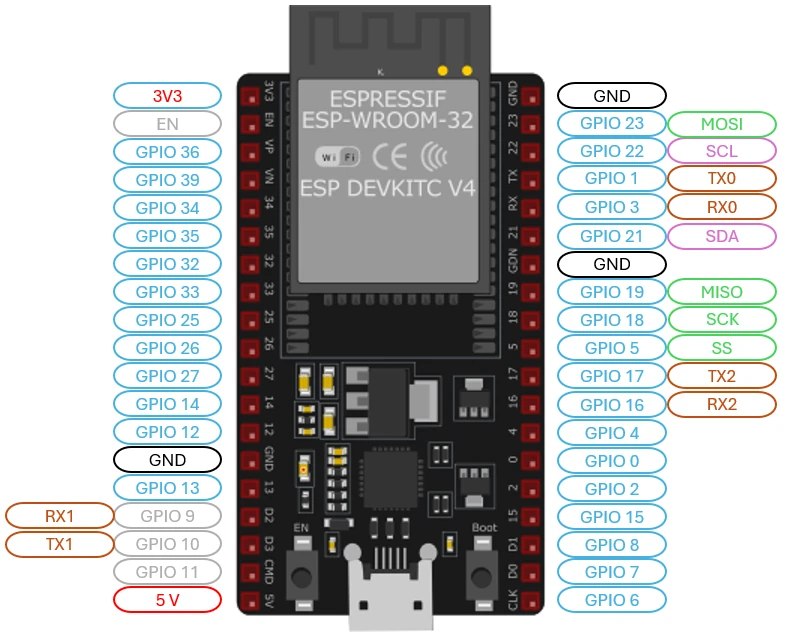
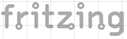
The most important functions of the individual PINs are shown above.
Here is a brief description of the other PIN functions:
- Input-Pins: EN, GPIO 34, 35, 36, 39
- Touch: GPIO 32, 33, 27, 14, 12, 13, 15, 2, 0, 4
- PWM: all Output-PINs, except GPIO 34 bis 39
- HIGH at the BOOT: PINs 1, 3, 5, 14, 15 (If LEDs or relays, for example, are connected here, this leads to unwanted states!)
- DAC: GPIO 25, 26 (Digital to analog converter)
- ADC: GPIO 0, 2, 4, 12-15, 25-27, 32-39 (Analog to digital converter)
- Nicht nutzen: GPIO 6 – 11. These pins are internally connected to the flash
CPU and internal memory
- The ESP32-DOWD has a Dual-Core Xtensa 32-bit LX6 MCU
- Internal memory with 448 KB ROM for boot and core functions
- 520 KB SPAM for data and instructions
- The ESP32 has 8 kilobyte (KB) SRAM memory,
which is located in the RTC area. This memory area is referred to as RTC FAST Memory. This special memory area can be used to store data that is to be retained during the ESP32’s deep sleep mode. Deep sleep is an energy-saving mode in which the majority of the chip is switched off, but the RTC and the RTC FAST memory can remain active.
Here is a simple example code that shows how you can use the RTC FAST memory in the ESP32:
#include <esp_sleep.h> // Variable im RTC FAST Memory RTC_DATA_ATTR int bootCount = 0; void setup() { Serial.begin(115200); // Increasing the boot counter bootCount++; Serial.print("Boot count: "); Serial.println(bootCount); // Simulate a task, then go into deep sleep mode delay(1000); // Wait a second // Go into deep sleep mode for 10 seconds esp_sleep_enable_timer_wakeup(10 * 1000000); // 10 Seconds in Mikrosekunden esp_deep_sleep_start(); } void loop() { // This code is not executed because the ESP32 is in deep sleep mode }
In the example above, a variable bootCount is stored in the RTC FAST memory and each time the ESP32 wakes up, the counter is incremented. The ESP32 then goes back into deep sleep mode and the next time it wakes up, the value of bootCount is still available.
- 8 KB SRAM in RTC (Real-Time Clock):
The ESP32 has 8 kilobytes (KB) of SRAM memory, which is located in the RTC area. This memory area is referred to as RTC SLOW memory. In contrast to the RTC FAST memory, which is used by the main processor after wake-up, the RTC SLOW memory can be actively used by the co-processor during deep sleep mode. This makes it possible to perform certain tasks in energy-saving mode without waking up the main processor.
Here is a simple example code that shows how you can use the RTC SLOW memory in the ESP32:
// Simple function for demonstrating the co-processor (ULP) and RTC SLOW memory #include "esp_sleep.h" #include "driver/rtc_io.h" RTC_DATA_ATTR int counter = 0; // Variable in the RTC SLOW memory void setup() { Serial.begin(115200); delay(1000); // Short delay to stabilize the serial connection if (esp_sleep_get_wakeup_cause() == ESP_SLEEP_WAKEUP_ULP) { // The co-processor has triggered the wake-up Serial.println("Woken up by the ULP co-processor"); counter++; } else { // Normal start or waking up for another reason Serial.println("Normal boot or other wakeup source"); } Serial.print("Counter value: "); Serial.println(counter); // Here you could program the ULP to monitor a sensor, for example // Example: Configure ULP and go into deep sleep mode esp_sleep_enable_ulp_wakeup(); esp_deep_sleep_start(); } void loop() { // This code is not executed because the ESP32 is in deep sleep mode }
In this example, a variable counter is stored in the RTC SLOW memory and the co-processor can wake up the ESP32 from deep sleep mode, incrementing the counter. The main processor can then read the new value of counter when it wakes up.
eFuse memory
The ESP32 has 1 Kbit (kilobit) of eFuse memory. This memory is used to store permanent and unchangeable data that is important for the configuration and security of the chip.
256 bits for the system: Of the total of 1024 bits, 256 bits are reserved for the system. These bits are used for important information such as:
MAC-Address: The unique MAC address of the chip, which is used for network communication and device identification.
Chip-Configuration: Configuration data required to operate the chip.
768 Bits for customer applications: The remaining 768 bits are reserved for use by the user. These bits can be used for various applications, including:
Flash encryption: Storage of keys and configuration data required to encrypt the flash memory. This increases security by preventing access to the flash memory without the appropriate authorizations.
Chip-ID: Storing a unique identification number of the chip, which can be used for various applications, e.g. for authentication or identification of the device.
Description of external SPI flash memory
Both modules, ESP32-WROOM-32D and ESP32-WROOM-32U, integrate a 4 MB (megabyte) external SPI flash memory. This memory is used to store firmware, applications and data required by the ESP32.
Important points
- Integrated SPI flash memory:
- 4 MB Storage space: Both modules have a 4 MB SPI flash memory, which offers sufficient space for applications and data.
- External memory: The memory is external to the ESP32 chip, but integrated on the same module.
- Connection to GPIO pins:
- The SPI flash memory is connected to certain GPIO pins (General Purpose Input/Output) of the ESP32. These are the pins GPIO6, GPIO7, GPIO8, GPIO9, GPIO10 and GPIO11.
- GPIO-Pins as a communication interface: These pins are used to enable communication between the ESP32 chip and the SPI flash memory. SPI (Serial Peripheral Interface) is a communication protocol that is often used to connect microcontrollers to external peripherals such as flash memory.
- Limitations of the GPIO pins:
- Cannot be used as regular GPIOs: The six pins mentioned (GPIO6 to GPIO11) cannot be used as normal GPIOs. This means that they cannot be used for other purposes such as reading sensors or controlling LEDs.
- Reserved for SPI flash: These pins are reserved exclusively for communication with the SPI flash memory and should not be configured for other tasks.
Why is this important?
- Understanding the pin assignment: If you are developing a project with the ESP32-WROOM-32D or ESP32-WROOM-32U, it is important to know which pins are available and which are reserved. This will help you avoid configuration conflicts and ensure that your project runs smoothly.
- Storage management: The external SPI flash memory provides additional storage space for larger firmware and data beyond the internal memory of the ESP32. This is particularly useful for more complex applications that require more memory.
Clock frequency: 40 MHz
The above information is not complete, but should be sufficient for a large part of your projects and should also encourage you to continue working with the ESP32.
If you have any questions or suggestions, please use the comment function and give me feedback.