In this DIYTechAdventure, I will show you how you can use a NodeMCU to make an HTTP request via WLAN and write the data received to a database on a web server. The NodeMCU is a microcontroller based on the ESP8266, which is ideal for IoT projects.
The ESP8266 articles are intended to bring us into the WiFi. Feel free to read up if you are unsure.
Introduction to HTTP requests
HTTP (Hypertext Transfer Protocol) is the protocol that enables the exchange of data over the Internet. It forms the basis for communication between web browsers and web servers.
HTTP requests consist of various components, including:
- Request-Line: This is the first line of an HTTP request and contains the method (GET, POST, PUT, DELETE, etc.), the URL and the HTTP version.
- Headers: These lines provide additional information about the request, such as the user agent, content type and much more.
- Body: The body contains the data to be sent to the server, especially for POST requests.
HTTP-Methods
- GET: Requests data from a server. The data is transmitted in the URL.
- POST: Sends data to a server, often to create new resources. The data is transmitted in the body of the request.
- PUT: Updates existing resources with the data sent.
- DELETE: Deletes a resource on the server.
GET /index.html HTTP/1.1 Host: www.example.com User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64) Accept: text/html
Now that you have a basic understanding of HTTP requests, let’s see how to make them with a NodeMCU and write data to a database on a web server.
Prerequisites
- A NodeMCU (ESP8266) -> you can get one cheaply at AzDelivery or Aliexpress
- Access to a WiFi network
- A web server with a database (e.g. MySQL)
- Basic knowledge of Arduino programming and SQL
Step 1: Set up NodeMCU
- Install the Arduino IDE:
Make sure that you have installed the Arduino IDE on your computer. Add the ESP8266 board by adding the URL http://arduino.esp8266.com/stable/package_esp8266com_index.json under “Additional board manager URLs” in the Arduino IDE preferences and then search for and install esp8266 under “Tools” -> “Board” -> “Board management”.
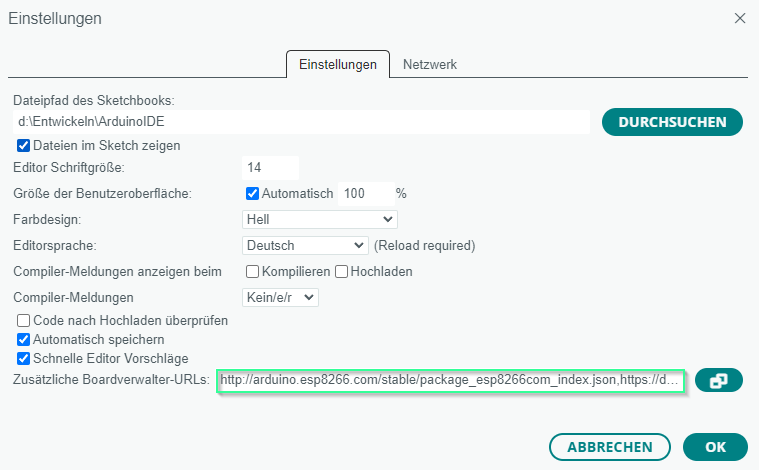
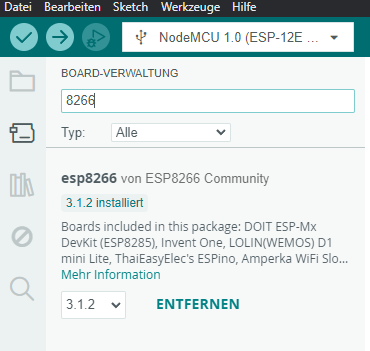
2. Install libraries:
You need the ESP8266WiFi and ESP8266HTTPClient libraries. You can install these via the library manager in the Arduino IDE.
Step 2: Connect to the WLAN
Create a new sketch and add the following code to connect the NodeMCU to your WLAN:
// This code is also used in the next step, you do not need to copy it #include <ESP8266WiFi.h> const char* ssid = "your_SSID"; const char* password = "your_PASSWORT"; void setup() { Serial.begin(115200); WiFi.begin(ssid, password); while (WiFi.status() != WL_CONNECTED) { delay(500); Serial.print("."); } Serial.println(""); Serial.println("WiFi connected"); Serial.print("IP-Adresse: "); Serial.println(WiFi.localIP()); } void loop() { // Nothing is needed here }
// these two constants record your WLAN name and password
const char* ssid = “your_SSID”;
const char* password = “your_PASSWORT”;
// Serial interface for outputting the print instructions
// Initialize WiFi
Serial.begin(115200);
WiFi.begin(ssid, password);
//Run through loop -> As long as WiFi.status is set to not connected -> Wait 500 ms -> Output a dot as a progress indicator
while (WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(“.”);
The IP address received from the WLAN router when establishing the connection is then output with serial.print.
Step 3: Send HTTP POST request
Now we add the code to send an HTTP POST request and transmit the data to the web server:
#include <ESP8266WiFi.h> #include <ESP8266HTTPClient.h> const char* ssid = "your WiFi Name"; const char* password = "your WLAN Passwort"; const char* serverName = "http://Your server/receive_script.php"; // URL to your PHP script void setup() { Serial.begin(115200); WiFi.begin(ssid, password); while (WiFi.status() != WL_CONNECTED) { delay(500); Serial.print("."); } Serial.println(""); Serial.println("WiFi connected"); Serial.print("IP-Adresse: "); Serial.println(WiFi.localIP()); if (WiFi.status() == WL_CONNECTED) { WiFiClient client; // WiFiClient-Create object HTTPClient http; http.begin(client, serverName); // Transfers the WiFiClient to HTTPClient http.addHeader("Content-Type", "application/x-www-form-urlencoded"); String postData = "name=NodeMCU&wert=123"; // Example data int httpResponseCode = http.POST(postData); if (httpResponseCode > 0) { String response = http.getString(); Serial.println(httpResponseCode); Serial.println(response); } else { Serial.print("Error on sending POST: "); Serial.println(httpResponseCode); } http.end(); } } void loop() { // Nothing is needed here }
In this code:
- WiFiClient client; erstellt eine Instanz der WiFiClient-Klasse.
- HTTPClient http; creates an instance of the HTTPClient class.
- http.begin(client, serverName); initializes the connection to the server with the specified URL.
- http.addHeader(“Content-Type”, “application/x-www-form-urlencoded”); adds a header that specifies the content type of the request.
- http.POST(postData); sends a POST request with the specified data to the server and returns the HTTP response code.
- The feedback is then output by the server
- http.end(); terminates the installed client
Step 4: PHP script on the server
Create a PHP script on your web server (e.g. empfangsscript.php) that writes the received data to the database:
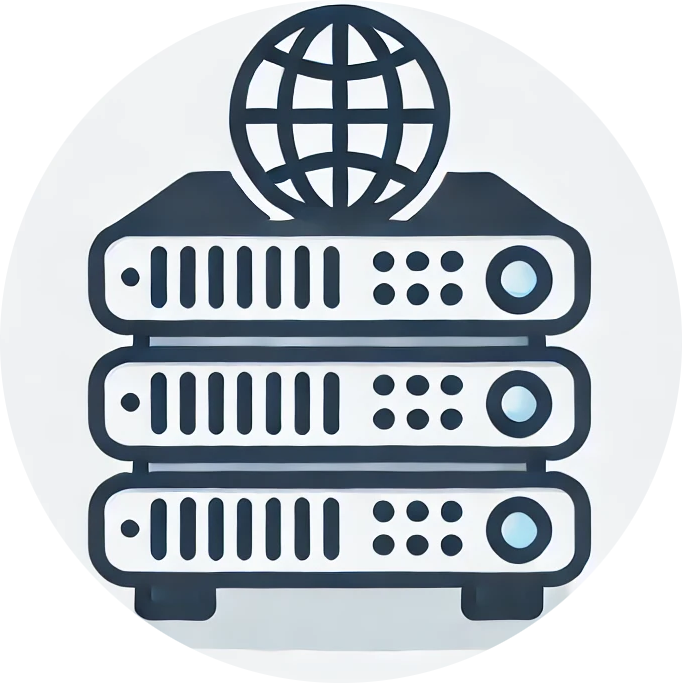
<?php // Provide login data for the database connection $servername = "localhost"; $username = "your_db_username"; $password = "your_db_passwort"; $dbname = "your_db_name"; // Establishing a connection to the database $conn = new mysqli($servername, $username, $password, $dbname); // Check connection if ($conn->connect_error) { die("Connection failed: " . $conn->connect_error); } // Reading data from the POST request $name = $_POST['name']; $wert = $_POST['wert']; // SQL query to insert the data $sql = "INSERT INTO deine_tabelle (name, wert) VALUES ('$name', '$wert')"; // Check whether the data has been written to the database if ($conn->query($sql) === TRUE) { echo "New record created successfully"; } else { echo "Error: " . $sql . "<br>" . $conn->error; } // Close database connection again $conn->close(); ?>
The above scripts show you the basic procedure for sending data to a web server.
But now to the output of the script’s print instructions:
18:57:46.487 -> WiFi connected
18:57:46.487 -> IP-Adresse: xxx.xxx.xx.xx
18:57:46.583 -> 200
18:57:46.583 -> New record created successfully
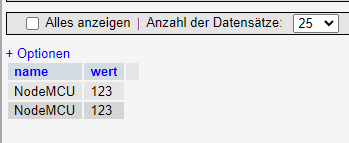
The data records have been transferred to the database without any problems.
I would say – goal achieved!
In this DIYTechAdventure, you have basically learned how to send an HTTP request with a NodeMCU via WLAN and save the data in a database on a web server. This knowledge forms the basis for many exciting IoT projects in which data from the real world is collected and processed.
In the next adventure, I will show you specifically how to send temperature data. In further posts, I’ll also show you how to proceed if you don’t have a web server.
Good luck with your projects and happy coding!