In this DIYTechAdventure, I will show you how you can use an ESP32 to make an HTTP request via WLAN and write the sensor data received from the SHT31 to a database on a web server. The ESP is a microcontroller that is ideal for IoT projects.
The articles ESP8266 in the WiFi and Temperature measurement with the SH3x should serve as a basic education. Feel free to read up if you are unsure.
Be sure to take a look at the basics of sending data to a web server. I will not go into the connection to the WLAN here.
As a learning effect, I have described the possibility of securing inserts to the database in this DIYTechAdventure.
Prerequisites
- A NodeMCU (ESP8266) -> you can get one cheaply at AzDelivery or Aliexpress
- You can get an SHT31-> cheaply from Aliexpress
- Access to a WiFi network
- A web server with a database (e.g. MySQL)
- Basic knowledge of Arduino programming and SQL
Here again is the circuit showing how an I2C bus is connected
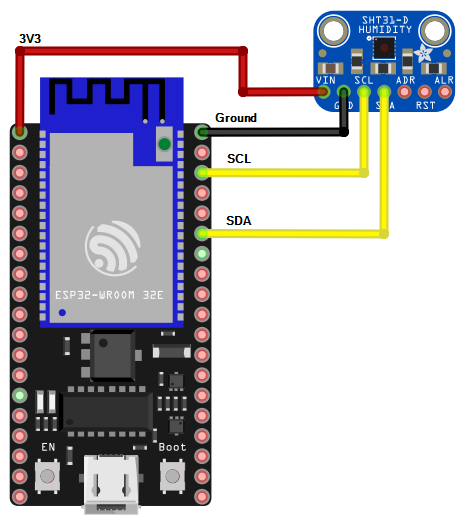
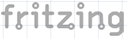
Code for establishing a WLAN connection, reading out the sensor data and sending it to a web server
//Code Eckhard Gerwien - This code is freely available // Include the required libraries #include <WiFi.h> #include <HTTPClient.h> #include <Wire.h> #include <SPI.h> #include <Adafruit_SHT31.h> // WiFi settings const char* ssid = "your_SSID"; const char* password = "your_PASSWORT"; // Webserver-URL const char* serverName = "http://your server.com/receive_script.php"; // Creating an object for the SHT31 sensor Adafruit_SHT31 sht31 = Adafruit_SHT31(); void setup() { // Initialization of serial communication for debugging Serial.begin(115200); // Initialization of the I2C bus Wire.begin(); // Initialization of the SHT31 sensor if (!sht31.begin(0x44)) { // 0x44 is the I2C address of the SHT31 Serial.println("Could not find SHT31, check the wiring!"); while (1) delay(1); } Serial.println("SHT31 Sensor found!"); // Establishing a connection to the WiFi WiFi.begin(ssid, password); Serial.print("Connect with WiFi"); while (WiFi.status() != WL_CONNECTED) { delay(500); Serial.print("."); } //Outputs to the serial interface Serial.println(""); Serial.println("WiFi connected"); Serial.print("IP-Address: "); Serial.println(WiFi.localIP()); } //Code that is regularly executed in a loop void loop() { // Reading out the temperature float temperature = sht31.readTemperature(); // Reading out the humidity float humidity = sht31.readHumidity(); // Check whether the measured values are valid if (!isnan(temperature)) { // isnan checks whether the value is 'NaN' (Not a Number) Serial.print("Temperature: "); Serial.print(temperature); Serial.println(" °C"); } else { Serial.println("Error when reading out the temperature!"); } if (!isnan(humidity)) { Serial.print("Humidity: "); Serial.print(humidity); Serial.println(" %"); } else { Serial.println("Error when reading out the humidity!"); } // Sending the data to the web server if (WiFi.status() == WL_CONNECTED) { HTTPClient http; http.begin(serverName); http.addHeader("Content-Type", "application/x-www-form-urlencoded"); // Transferring the measured values to the string for sending String postData = "temperature=" + String(temperature) + "&humidity=" + String(humidity); int httpResponseCode = http.POST(postData); if (httpResponseCode > 0) { String response = http.getString(); Serial.println(httpResponseCode); Serial.println(response); } else { Serial.print("Error on sending POST: "); Serial.println(httpResponseCode); } http.end(); } else { Serial.println("WiFi not connected"); } // Waiting time of 10 seconds before the next measurement delay(10000); }
In this code:
- HTTPClient http; creates an instance of the HTTPClient class.
- http.begin(client, serverName); initializes the connection to the server with the specified URL.
- http.addHeader(“Content-Type”, “application/x-www-form-urlencoded”); adds a header that specifies the content type of the request.
- http.POST(postData); sends a POST request with the specified data to the server and returns the HTTP response code.
- The feedback is then output by the server
- http.end(); terminates the installed client
PHP script on the server
Create a PHP script on your web server (e.g. empfangsscript.php) that writes the received data to the database:
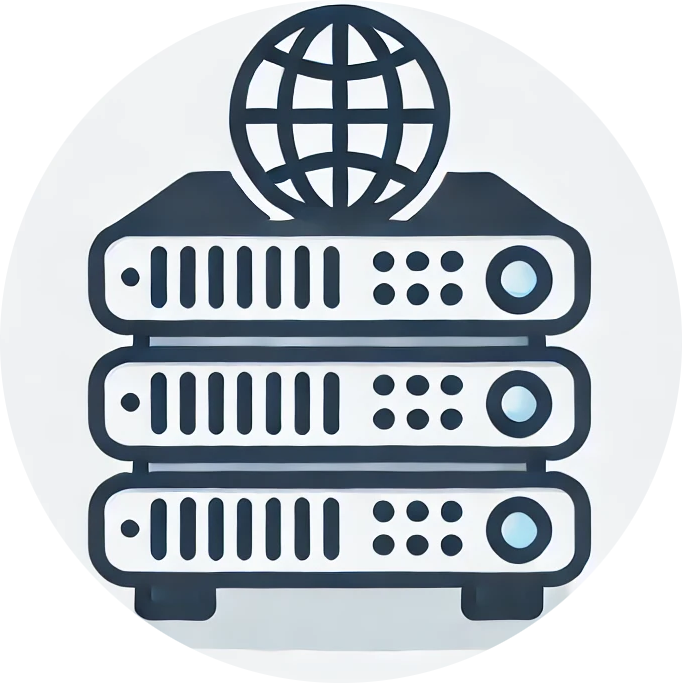
<?php // Provide login data for the database connection $servername = "########"; $username = "#######"; $password = "#######"; $dbname = "######"; // Establishing a connection to the database $conn = new mysqli($servername, $username, $password, $dbname); // Check connection if ($conn->connect_error) { die("Connection failed: " . $conn->connect_error); } // Read data from the POST request $temperature = $_POST['temperature']; $humidity = $_POST['humidity']; // Prepare SQL query to insert the data $sql = $conn->prepare("INSERT INTO projekt (temperature, humidity) VALUES (?, ?)"); $sql->bind_param("ss", $temperature, $humidity); // "ss" means that two string values are bound // Check whether the data has been written to the database if ($sql->execute()) { echo "New record created successfully"; } else { echo "Error: " . $sql->error; } // Close database connection again $sql->close(); $conn->close(); ?>
Line 3 – 6: Variables for the database connection
Line 9: Variables agreed above are required for the connection string
Line 12 – 14: If the connection to the database fails, terminate the script
Line 17 – 18: Transfer data from the post-request
Line 21 – 22: Here I execute a parameter binding or prepared statements. Both terms describe the process of securing SQL inserts. Parameter binding is used to separate the input values from the actual SQL code, which helps to prevent SQL injection attacks.
Line 25 – 28: Output of the result of our efforts
Line 32 – 33: Break down connections
Die Ausgabe auf der seriellen Schnittstelle
18:46:54.818 -> Temperature: 26.29 °C
18:46:54.818 -> Humidityt: 43.49 %
18:46:54.914 -> 200
18:46:54.914 -> New record created successfully
18:47:04.947 -> Temperature: 26.30 °C
18:47:04.978 -> Humidity: 43.46 %
18:47:05.043 -> 200
18:47:05.043 -> New record created successfully
18:47:15.103 -> Temperature: 26.27 °C
18:47:15.103 -> Humidity: 43.38 %
18:47:17.771 -> 200
18:47:17.771 -> New record created successfully
18:47:27.830 -> Temperature: 26.29 °C
18:47:27.830 -> Humidity: 43.51 %
18:47:27.894 -> 200
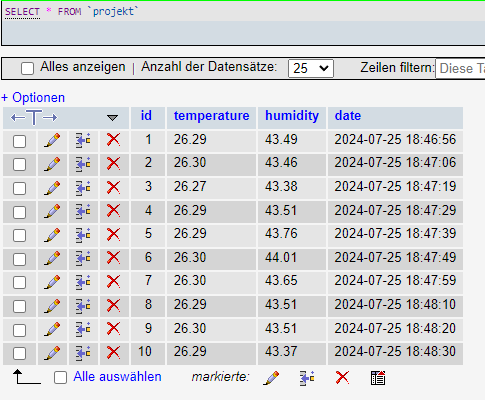
The data records have been transferred to the database without any problems. Instead of date, the column could contain the correct timestamp. But that’s ok.
I would say – goal achieved!
In this DIYTechAdventure, you also learned how to send an HTTP request with a NodeMCU via WLAN and save the sensor data in a database on a web server with SQL parameter binding.
In further articles, I will also show you how to proceed if you do not have a web server.
And if you have any questions or suggestions, feel free to write a comment.
Good luck with your projects and happy coding!