For the sake of completeness, here is a brief overview of previous posts in which I described the following in detail:
Now the final step is to follow and we will connect our ESP32 to the WLAN and send the sensor data to our own web server and save it in the database, as described in the article Sending sensor data to the web server.
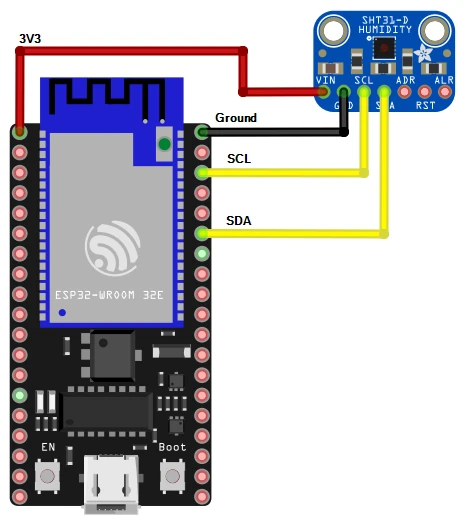
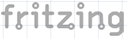
The prerequisite is the circuit as described in the article
Temperature measurement with the SH3x. It also describes exactly how to get the sketch below onto your ESP using the Arduino IDE.
Before you load the sketch onto the board, you need to find out the IP address of your computer.
You can check this on your router or open the cmd program on your computer and enter the command IPconfig. The address has a similar structure -> 192.xxx.xx.xx. Please enter this IP address in the variable const char* serverName after http:// instead of “deine_IP”. For the WLAN connection, the WiFi name and password must also be exchanged here. Important: The PC must be logged into the same WiFi network in which the ESP32 has logged in.
//Code Eckhard Gerwien - This code is freely available // Integration of the required libraries #include <WiFi.h> #include <HTTPClient.h> #include <Wire.h> #include <SPI.h> #include <Adafruit_SHT31.h> // WLAN-Einstellungen const char* ssid = "your_SSID"; const char* password = "your_PASSWORT"; // Webserver-URL const char* serverName = "http://Your_IP/sensordata/receive_script.php"; // Creating an object for the SHT31 sensor Adafruit_SHT31 sht31 = Adafruit_SHT31(); void setup() { // Initialization of serial communication for debugging Serial.begin(115200); // Initialization of the I2C bus Wire.begin(); // Initializing the SHT31 sensor if (!sht31.begin(0x44)) { // 0x44 is the I2C address of the SHT31 Serial.println("Could not find SHT31, check the wiring!"); while (1) delay(1); } Serial.println("SHT31 sensor found!"); // Establishing a connection to the WiFi WiFi.begin(ssid, password); Serial.print("Connect with WiFi"); while (WiFi.status() != WL_CONNECTED) { delay(500); Serial.print("."); } //Outputs to the serial interface Serial.println(""); Serial.println("Outputs to the serial interfaceOutputs to the serial interface"); Serial.print("IP-Address: "); Serial.println(WiFi.localIP()); } //Code that is regularly executed in a loop void loop() { // Reading the temperature float temperature = sht31.readTemperature(); // Reading the humidity float humidity = sht31.readHumidity(); // ÜCheck if the measured values are valid if (!isnan(temperature)) { // isnan checks whether the value is 'NaN' (Not a Number) Serial.print("Temperature: "); Serial.print(temperature); Serial.println(" °C"); } else { Serial.println("Error when reading out the temperature!"); } if (!isnan(humidity)) { Serial.print("Humidity: "); Serial.print(humidity); Serial.println(" %"); } else { Serial.println("Error when reading out the humidity!"); } // Sending the data to the web server if (WiFi.status() == WL_CONNECTED) { HTTPClient http; http.begin(serverName); http.addHeader("Content-Type", "application/x-www-form-urlencoded"); // Transferring the measured values to the string for sending String postData = "temperature=" + String(temperature) + "&humidity=" + String(humidity); int httpResponseCode = http.POST(postData); if (httpResponseCode > 0) { String response = http.getString(); Serial.println(httpResponseCode); Serial.println(response); } else { Serial.print("Error on sending POST: "); Serial.println(httpResponseCode); } http.end(); } else { Serial.println("WiFi not connected"); } // Wartezeit von 10 Sekunden vor der nächsten Messung delay(10000); }
Please download the sketch above to your ESP.
If you need to read it again, go to this Post.
Server and directory settings
Go to your xampp installation directory. There you will find the folder htdocs – please open it.
Create a new directory, e.g. sensordata. This directory contains all the files that are required for a website. In our case, add our php script (e.g. receive_script.php), which will receive the sensor data sent by the ESP32 and send it to the database.
Please note that the 4 variables at the beginning of the script are filled with your values.
If you have not made any settings to the rights under phpMyAdmin, the user name “root” and no password should be assigned. Then enter the DB name of your created database. In my case sensor data.
?php //PHP Script for extracting the data from the HTTP request and saving it in a database // Provide login data for the database connection $servername = "localhost"; $username = "########"; // your user - default root $password = "#######"; // your password - default none assigned $dbname = "sensordaten"; // your database name // Establishing a connection to the database $conn = new mysqli($servername, $username, $password, $dbname); // Check connection if ($conn->connect_error) { die("Connection failed: " . $conn->connect_error); } // Read data from the POST request $temperature = $_POST['temperature']; $humidity = $_POST['humidity']; // Prepare SQL query to insert the data $sql = $conn->prepare("INSERT INTO sht31_daten (temperature, humidity) VALUES (?, ?)"); $sql->bind_param("ss", $temperature, $humidity); // "ss" means that two string values are bound // Check whether the data has been written to the database if ($sql->execute()) { echo "New record created successfully"; } else { echo "Error: " . $sql->error; } // Close database connection again $sql->close(); $conn->close(); ?>
If everything went well, you should see the following. HTTP response code: 200 tells us -> OK! Everything worked.
11:57:12.089 -> SHT31 Sensor found!
11:57:12.188 -> Connect with WiFi…..
11:57:14.667 -> WiFi connected
11:57:14.667 -> IP-Address: 192.xxx.x.xxx
11:57:14.698 -> Temperature: 27.43 °C
11:57:14.731 -> Humidity: 51.28 %
11:57:14.827 -> HTTP Response code: 200
11:57:14.827 -> New record created successfully
11:57:24.870 -> Temperature: 27.42 °C
11:57:24.870 -> Humidity: 51.60 %
11:57:24.934 -> HTTP Response code: 200
11:57:24.934 -> New record created successfully
If something goes wrong, you will receive an error message. Here it is important to understand where something is going wrong. We have received the temperature and humidity. The sketch on the ESP32 seems to be running. Please check whether the correct IP address has been entered. It is also possible to enter the link http://Deine_IP/sensordaten/empfangsscript.php directly into the address field in the browser. This will tell you whether the script can be accessed.
11:57:18.089 -> SHT31 Sensor found!
11:57:18.188 -> Connect with WiFi…..
11:57:18.667 -> WiFi connected
11:57:18.667 -> IP-Address: 192.xxx.x.xxx
11:57:19.338 -> Temperature: 27.28 °C
11:57:19.338 -> Humidity: 50.93 %
11:57:24.318 -> Error on sending POST: -1
Go back to your created database in phpMyAdmin and you should also see the saved values in the database.
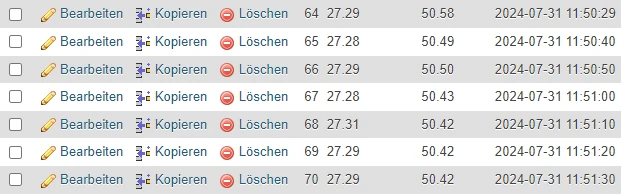
In this DIYTechAdventure you have seen how sensor data can be sent from a sensor to your PC via your WLAN router. With a database server like xampp, you are independent of the web and can develop and extensively test your projects. Let me know in the comments how you got on with this post. That way I can also learn how to design posts better.